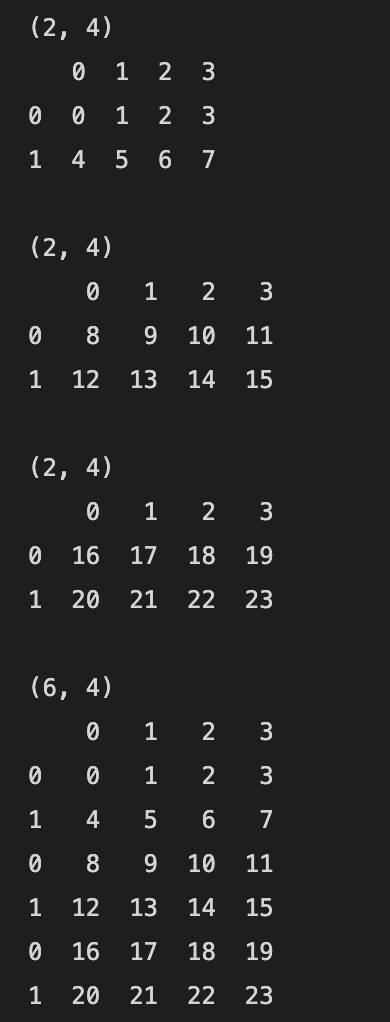
“10 minutes to pandas” 문서를 따라해보며 작성했습니다.
https://pandas.pydata.org/pandas-docs/stable/user_guide/10min.html
2022. 02. 02 최초작성
2022. 03. 20
연결하기
concat 메소드를 사용하여 열방향으로 DataFrame을 연결합니다.
import numpy as np import pandas as pd df1 = pd.DataFrame(np.arange(8).reshape(2,4)) print(df1.shape) print(df1) print() df2 = pd.DataFrame(np.arange(8,16).reshape(2,4)) print(df2.shape) print(df2) print() df3 = pd.DataFrame(np.arange(16,24).reshape(2,4)) print(df3.shape) print(df3) print() df = pd.concat([df1, df2, df3]) print(df.shape) print(df) print() |
concat 메소드를 사용하여 행방향으로 DataFrame을 연결합니다.
import numpy as np import pandas as pd df1 = pd.DataFrame(np.arange(8).reshape(2,4)) print(df1.shape) print(df1) print() df2 = pd.DataFrame(np.arange(8,16).reshape(2,4)) print(df2.shape) print(df2) print() df = pd.concat([df1, df2], axis=1) print(df.shape) print(df) |
append 메소드 사용하여 DataFrame을 연결하는 방법입니다. 열 방향으로 연결됩니다.
import numpy as np import pandas as pd df1 = pd.DataFrame(np.arange(8).reshape(2,4)) print(df1.shape) print(df1) print() df2 = pd.DataFrame(np.arange(8,16).reshape(2,4)) print(df2.shape) print(df2) print() result = df1.append(df2) print(result.shape) print(result) |
경고문으로 append 대신에 concat을 사용하라는 메시지가 다음처럼 출력됩니다.
/var/folders/hw/kk3pjps15015_hk0lq9wfjym0000gn/T/ipykernel_23042/4132674957.py:15: FutureWarning: The frame.append method is deprecated and will be removed from pandas in a future version. Use pandas.concat instead.
result = df1.append(df2)
그룹핑(Grouping) 하기
지정한 열의 값을 기준으로 그룹핑합니다.
import numpy as np import pandas as pd df = pd.DataFrame( { "A": ["foo", "bar", "foo", "bar", "foo", "bar", "foo", "foo"], "B": ["one", "one", "two", "three", "two", "two", "one", "three"], "C": [ 1, 2, 3, 4, 5, 6, 7, 8], "D": [ -1, -2, -3, -4, -5, -6, -7, -8], } ) print(df) print() # A열을 기준으로 그룹핑한 결과를 살펴봅니다. print(df.groupby("A").apply(print)) print() # A열을 기준으로 그룹핑한 결과에 sum() 메소드를 적용합니다. print(df.groupby("A").sum()) print() # B열을 기준으로 그룹핑한 결과를 살펴봅니다. print(df.groupby("B").apply(print)) print() # B열을 기준으로 그룹핑한 결과에 sum() 메소드를 적용합니다. print(df.groupby("B").sum()) |
두 개의 열을 기준으로 그룹핑합니다.
import numpy as np import pandas as pd df = pd.DataFrame( { "A": ["foo", "bar", "foo", "bar", "foo", "bar", "foo", "foo"], "B": ["one", "one", "two", "three", "two", "two", "one", "three"], "C": [ 1, 2, 3, 4, 5, 6, 7, 8], "D": [ -1, -2, -3, -4, -5, -6, -7, -8], } ) print(df) print() # A열, B열 기준으로 그룹핑한 결과를 살펴봅니다. print(df.groupby(["A", "B"]).apply(print)) print() # A열, B열 기준으로 그룹핑한 결과에 sum() 메소드를 적용합니다. print(df.groupby(["A", "B"]).sum()) -- |
Pandas 강좌 1 - Pandas 객체 생성
https://webnautes.tistory.com/1957
Pandas 강좌 2 - 데이터 보는 방법
https://webnautes.tistory.com/1958
Pandas 강좌 3 - 데이터 선택하는 방법
https://webnautes.tistory.com/1959
Pandas 강좌 4 - 연산(Operations)
https://webnautes.tistory.com/1960
Pandas 강좌 5 - 연결 및 그룹핑
https://webnautes.tistory.com/1961
Pandas 강좌 6 - 시계열(Time series)
https://webnautes.tistory.com/1962
Pandas 강좌 7 - 그래프 그리기(Plotting)
https://webnautes.tistory.com/1963
Pandas 강좌 8 - Pandas에서 CSV, HDF5, Excel로 저장 및 읽기
https://webnautes.tistory.com/1964
Pandas 강좌 9 - 결측치(Missing data)
https://webnautes.tistory.com/1965
'Python > Pandas' 카테고리의 다른 글
Pandas 강좌 7 - 그래프 그리기(Plotting) (0) | 2023.10.12 |
---|---|
Pandas 강좌 6 - 시계열(Time series) (0) | 2023.10.12 |
Pandas 강좌 4 - 연산(Operations) (0) | 2023.10.12 |
Pandas 강좌 2 - 데이터 보는 방법 (0) | 2023.10.12 |
Pandas 강좌 1 - Pandas 객체 생성 (0) | 2023.10.12 |
시간날때마다 틈틈이 이것저것 해보며 블로그에 글을 남깁니다.
블로그의 문서는 종종 최신 버전으로 업데이트됩니다.
여유 시간이 날때 진행하는 거라 언제 진행될지는 알 수 없습니다.
영화,책, 생각등을 올리는 블로그도 운영하고 있습니다.
https://freewriting2024.tistory.com
제가 쓴 책도 한번 검토해보세요 ^^
그렇게 천천히 걸으면서도 그렇게 빨리 앞으로 나갈 수 있다는 건.
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!