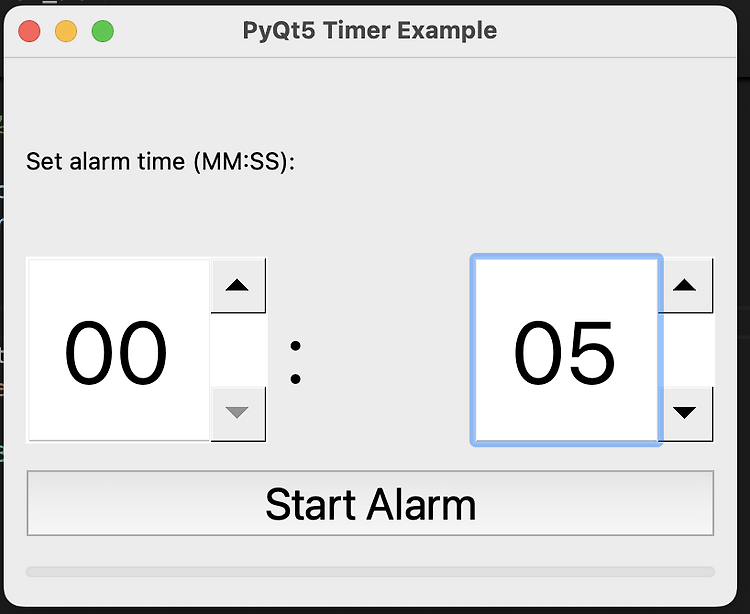
pyQt5을 사용하여 만든 간단한 알람 시계Qt/PyQt5 강좌2024. 9. 10. 21:43
Table of Contents
반응형
pyQt5을 사용하여 만든 간단한 알람 시계입니다.
2024. 8. 20 최초작성
2024. 9. 10 시 추가
알람이 울릴 시분초를 설정한 후 버튼을 클릭합니다.
설정한 시간이 00:00:00에 가까워지면서 파란색 프로그레스바가 이동합니다.
00: 00:00이 되면 메시지 박스가 보이게 됩니다.
전체 코드입니다.
import sys from PyQt5.QtWidgets import QApplication, QMainWindow, QMessageBox, QVBoxLayout, QWidget, QPushButton, QSpinBox, QLabel, QHBoxLayout, QProgressBar from PyQt5.QtCore import QTimer, Qt class CustomSpinBox(QSpinBox): def __init__(self, parent=None, max_value=59): super().__init__(parent) self.setAlignment(Qt.AlignCenter) self.setRange(0, max_value) def textFromValue(self, value): return f"{value:02d}" # 두 자리 숫자로 표시 def stepBy(self, steps): value = self.value() + steps if value > self.maximum(): value = self.minimum() elif value < self.minimum(): value = self.maximum() self.setValue(value) def initStyleOption(self, option): super().initStyleOption(option) option.displayAlignment = Qt.AlignCenter class MainWindow(QMainWindow): def __init__(self): super().__init__() self.initUI() def initUI(self): self.setWindowTitle("PyQt5 Timer Example") self.setGeometry(100, 100, 500, 300) self.main_widget = QWidget(self) self.setCentralWidget(self.main_widget) self.layout = QVBoxLayout(self.main_widget) self.label = QLabel("Set alarm time (HH:MM:SS):", self) self.layout.addWidget(self.label) self.time_layout = QHBoxLayout() # 시간 SpinBox 추가 self.hour_spinbox = CustomSpinBox(self, max_value=23) self.hour_spinbox.setStyleSheet(""" QSpinBox { font-size: 48px; width: 100px; height: 100px; } QSpinBox::up-button, QSpinBox::down-button { width: 30px; height: 30px; } QSpinBox::up-arrow, QSpinBox::down-arrow { width: 15px; height: 15px; } """) self.time_layout.addWidget(self.hour_spinbox) self.colon_label1 = QLabel(":", self) self.colon_label1.setStyleSheet("QLabel { font-size: 48px; }") self.time_layout.addWidget(self.colon_label1) self.minute_spinbox = CustomSpinBox(self) self.minute_spinbox.setStyleSheet(self.hour_spinbox.styleSheet()) self.time_layout.addWidget(self.minute_spinbox) self.colon_label2 = QLabel(":", self) self.colon_label2.setStyleSheet("QLabel { font-size: 48px; }") self.time_layout.addWidget(self.colon_label2) self.second_spinbox = CustomSpinBox(self) self.second_spinbox.setStyleSheet(self.hour_spinbox.styleSheet()) self.time_layout.addWidget(self.second_spinbox) self.layout.addLayout(self.time_layout) self.start_button = QPushButton("Start Alarm", self) self.start_button.setStyleSheet("QPushButton { font-size: 24px; }") self.start_button.clicked.connect(self.toggle_alarm) self.layout.addWidget(self.start_button) self.progress_bar = QProgressBar(self) self.progress_bar.setRange(0, 100) self.progress_bar.setValue(0) self.layout.addWidget(self.progress_bar) self.timer = QTimer(self) self.timer.setInterval(1000) self.timer.timeout.connect(self.update_timer) def toggle_alarm(self): if self.timer.isActive(): self.timer.stop() self.start_button.setText("Start Alarm") self.progress_bar.setValue(0) else: self.start_alarm() self.start_button.setText("Stop Alarm") def start_alarm(self): self.hours = self.hour_spinbox.value() self.minutes = self.minute_spinbox.value() self.seconds = self.second_spinbox.value() total_seconds = (self.hours * 3600) + (self.minutes * 60) + self.seconds if total_seconds > 0: self.total_seconds = total_seconds self.elapsed_seconds = 0 self.timer.start() else: QMessageBox.warning(self, "Invalid Time", "Please set a time greater than 0 seconds.") def update_timer(self): if self.seconds == 0: if self.minutes == 0: if self.hours == 0: self.timer.stop() self.show_alert() self.start_button.setText("Start Alarm") self.progress_bar.setValue(0) return else: self.hours -= 1 self.minutes = 59 self.seconds = 59 else: self.minutes -= 1 self.seconds = 59 else: self.seconds -= 1 self.hour_spinbox.setValue(self.hours) self.minute_spinbox.setValue(self.minutes) self.second_spinbox.setValue(self.seconds) self.elapsed_seconds += 1 progress = int((self.elapsed_seconds / self.total_seconds) * 100) self.progress_bar.setValue(progress) def show_alert(self): msg = QMessageBox() msg.setIcon(QMessageBox.Information) msg.setText("This is a timer alert!") msg.setWindowTitle("Alert") msg.setStandardButtons(QMessageBox.Ok) msg.exec_() if __name__ == '__main__': app = QApplication(sys.argv) mainWindow = MainWindow() mainWindow.show() sys.exit(app.exec_()) |
반응형
'Qt > PyQt5 강좌' 카테고리의 다른 글
PyQt5로 구현한 버튼으로 스크롤 가능한 리스트뷰(QListWidget) 예제 (0) | 2024.09.29 |
---|---|
pyQt5에서 레이아웃을 사용하는 이유 (0) | 2024.09.22 |
PyQt5 QDateEdit 사용 예제 (0) | 2024.09.04 |
QTableWidget의 컬럼 너비(크기)를 수동으로 조정하기 (1) | 2024.09.03 |
PyQt5 라벨 사용 예제 - QLabel, QFont, StyleSheet (4) | 2024.09.02 |